Get started¶
Note
Please ensure you have a working installation before proceeding with this tutorial.
Hello Tesseract¶
The tesseract
command line application provides tools to build Tesseracts as Docker containers from tesseract_api.py
files. Here, we’ll use it to build and invoke a simple Tesseract that greets you by name.
Building your first Tesseract¶
Download the Tesseract examples
and run the following command from where you unpacked the archive:
$ tesseract build examples/helloworld
[i] Building image ...
[i] Built image sha256:95e0b89e9634, ['helloworld:latest']
Tip
Having issues building Tesseracts? Check out common issues with the installation process.
Congratulations! You’ve just built your first Tesseract, which is now available as a Docker image on your system.
Running your Tesseract¶
You can interact with any built Tesseract via the command line interface (CLI), the REST API, or through the Python API (which uses CLI / REST under the hood). Try the commands below to see your Tesseract in action:
$ tesseract run helloworld apply '{"inputs": {"name": "Osborne"}}'
{"greeting":"Hello Osborne!"}
$ tesseract serve -p 8080 helloworld
[i] Waiting for Tesseract containers to start ...
[i] Container ID: 2587deea2a2efb6198913f757772560d9c64cf8621a6d1a54aa3333a7b4bcf62
[i] Name: tesseract-uum375qt6dj5-sha256-9by9ahsnsza2-1
[i] Entrypoint: ['tesseract-runtime', 'serve']
[i] View Tesseract: http://127.0.0.1:56489/docs
[i] Docker Compose Project ID, use it with 'tesseract teardown' command: tesseract-u7um375qt6dj5
{"project_id": "tesseract-u7um375qt6dj5", "containers": [{"name": "tesseract-uum375qt6dj5-sha256-9by9ahsnsza2-1", "port": "8080"}]}%
$ # The port at which your Tesseract will be served is random if `--port` is not specified;
$ # specify the one you received from `tesseract serve` output in the next command.
$ curl -d '{"inputs": {"name": "Osborne"}}' \
-H "Content-Type: application/json" \
http://127.0.0.1:8080/apply
{"greeting":"Hello Osborne!"}
$ tesseract teardown tesseract-9hj8fyxrx073
[i] Tesseracts are shutdown for Project name: tesseract-9hj8fyxrx073
>>> from tesseract_core import Tesseract
>>>
>>> with Tesseract.from_image("helloworld") as helloworld:
>>> helloworld.apply({"name": "Osborne"})
{'greeting': 'Hello Osborne!'}
Now, have a look at the (auto-generated) CLI and REST API docs for your Tesseract:
$ tesseract run helloworld --help
$ tesseract apidoc helloworld
[i] Waiting for Tesseract containers to start ...
[i] Serving OpenAPI docs for Tesseract helloworld at http://127.0.0.1:59569/docs
[i] Press Ctrl+C to stop
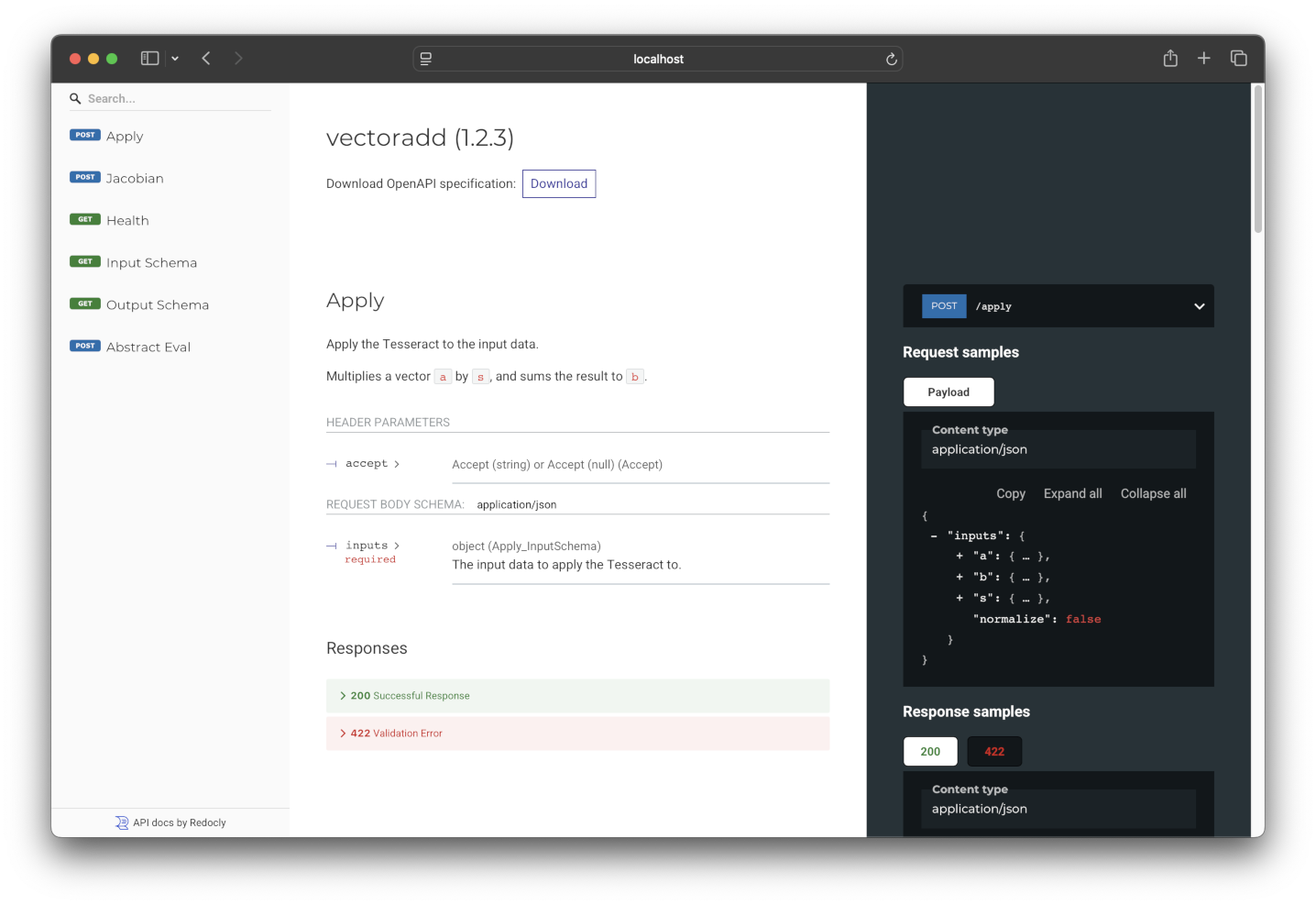
The OpenAPI docs for the vectoradd
Tesseract, documenting its endpoints and valid inputs / outputs.¶
Let’s peek under the hood¶
The folder passed to tesseract build
contains the files needed to build and run the Tesseract:
$ tree examples/helloworld
examples/helloworld
├── tesseract_api.py
├── tesseract_config.yaml
└── tesseract_requirements.txt
These files are all that’s needed to define a Tesseract.
tesseract_api.py
¶
The tesseract_api.py
file defines the Tesseract’s input and output schemas, and the functions that are being called when we invoke tesseract run <funcname>
. These are,
apply
, abstract_eval
, jacobian
, jacobian_vector_product
, and vector_jacobian_product
(see endpoints). Out of all of the endpoints you
can implement, only apply
is required for a Tesseract to work.
class InputSchema(BaseModel):
name: str = Field(description="Name of the person you want to greet.")
class OutputSchema(BaseModel):
greeting: str = Field(description="A greeting!")
def apply(inputs: InputSchema) -> OutputSchema:
"""Greet a person whose name is given as input."""
return OutputSchema(greeting=f"Hello {inputs.name}!")
Tip
For a Tesseract that has all optional endpoints implemented, check out the Univariate example.
tesseract_config.yaml
¶
tesseract_config.yaml
contains the Tesseract’s metadata, such as its name, description, version, and build configuration.
name: "helloworld"
version: "1.0.0"
description: "A sample Python app"
build_config:
# Base image to use for the container, must be Ubuntu or Debian-based
# base_image: "debian:bookworm-slim"
# Platform to build the container for. In general, images can only be executed
# on the platform they were built for.
# target_platform: "native"
# Additional packages to install in the container (via apt-get)
# extra_packages:
# - package_name
# Data to copy into the container, relative to the project root
# package_data:
# - [path/to/source, path/to/destination]
# Additional Dockerfile commands to run during the build process
# custom_build_steps:
# - |
# RUN echo "Hello, World!"
tesseract_requirements.txt
¶
tesseract_requirements.txt
lists the Python packages required to build and run the Tesseract.
Note
The tesseract_requirements.txt
file is optional. In fact, tesseract_api.py
is free to invoke functions that are not written in Python at all. In this case, use the build_config
section in tesseract_config.yaml
to provide data files and install the necessary dependencies.
# Add Python requirements like this:
# numpy==1.18.1
The journey continues…¶
Now, you’re ready to learn more, depending on your needs: